What constitutes an Advanced Lookup Filter?
An Advanced Lookup Filter represents a tailored filtering mechanism achieved via an Apex class. This customization enables enhanced flexibility in retrieving the desired dataset for a lookup field.
How do I Establish an Advanced Lookup?
Establishing an Advanced Lookup Filter involves crafting an Apex class that adheres to the Callable interface. Within this class, you have the freedom to define any necessary logic to fulfill your criteria. For more details about callable interface check here.
While creating an Apex Class for advanced lookup filter make sure:
- You use callable interface
- You implemented
public Object call(String action,Map<String, Object> args)
- Your class and methods are
global
Your private method
returns private static Map<String, String>
Here is a sample Apex class:
global class MyCustomLookupClass implements Callable {
private static Map<String, String> getLookupData(Map<String, Object> args){
Id myParameter = String.valueOf(args.get('myParameter'));
String searchKey = String.valueOf(args.get('bzb_searchKey')) + '%';
List<Account> accounts = [SELECT Id, Name FROM Account WHERE ParentId =:myParameter AND Name like :searchKey];
Map<String, String> result = new Map<String, String>();
for(Account acc: accounts){
result.put(acc.Id, acc.Name);
}
return result;
}
global Object call(String methodName, Map<String, Object> args) {
return getLookupData(args);
}
}
Available Parameters:
Below parameters are available while creating your custom apex class:
-
- bzb_connectionName: The unique connection name created for your org
-
- bzb_searchKey: The search key word entered from UI
-
- bzb_defaultValue: Default lookup value (if set to any value)
-
- bzb_objectApiName: Lookup reference object API Name
-
- bzb_displayFieldApiName: Lookup display field Api name (i.e: Name)
-
- bzb_reportFormId: The unique salesforce Id of the form
-
- bzb_formExternalId: The unique external Id of the form.
-
- Besides from above standard parameters., you can access any parameters you send as queryString in your form url.
What Comes Next? Once you’ve crafted and validated your new Apex class, simply input the name (including the namespace, if applicable) of the Apex class beneath the field, and you’re ready to proceed.
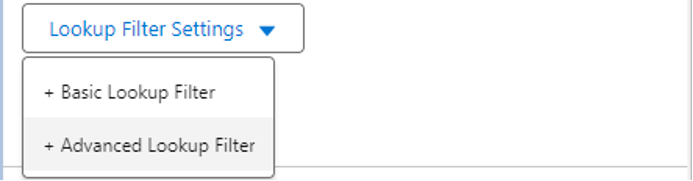
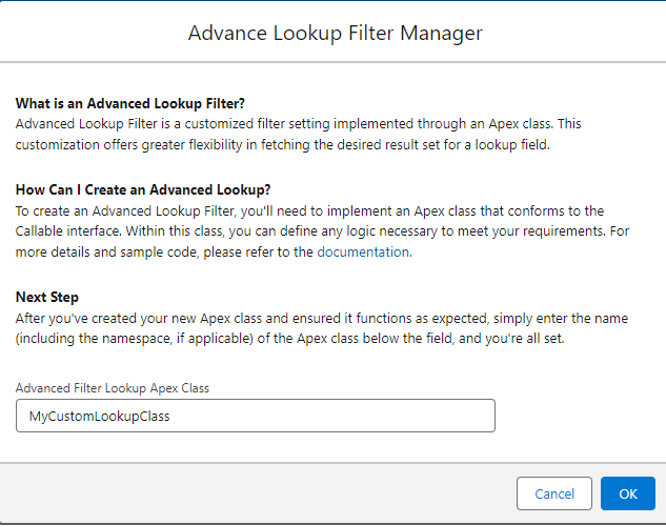